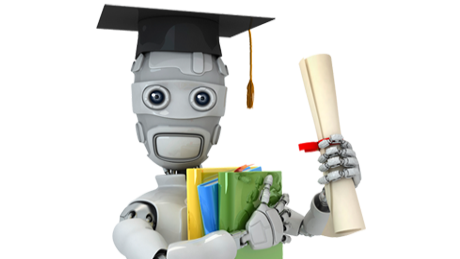
[Tensorflow] 07 MNIST data 학습하기
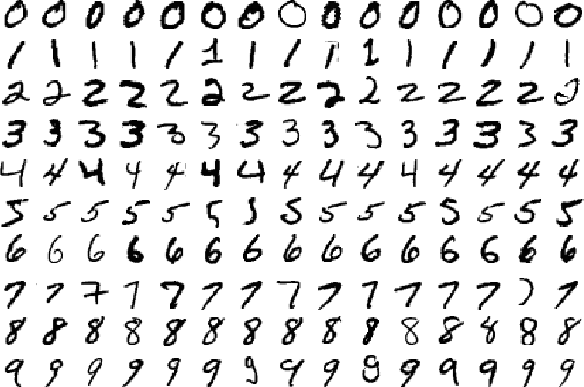
이론
위 그림이 MNIST Dataset입니다. 0~9 사이의 숫자를 손으로 쓴 데이터 집합입니다. 머신러닝을 통해 숫자를 구분시키는 학습을 시켜보려 합니다.
01) 어떻게…?
MNIST data는 위와 같이 28×28 pixel의 이미지, 다시 말해 784 pixel의 정보를 담고 있습니다. 데이터를 직접 들여다보지는 않았지만 검은색은 1.0, 흰색은 0, 회색은 0.5로 정리해놓은 형태일 것이라고 추측됩니다. [06 Softmax classification] 에서 동물들의 정보다 담긴 여러 데이터를 합쳐 하나의 동물 Label을 예측했던 것처럼 이번 학습에서는 784개의 데이터를 합쳐 하나의 숫자 Label을 예측해 보려 합니다.
02) Softmax Classification
위 설명대로 Softmax Classification 이론을 그대로 이용합니다.
Softmax Classification :: http://theyoonicon.com/06-softmax-classification-multinomial-classification/
03) Batch / Epoch
Batch ::
방대한 양의 데이터를 나눠서 불러들여오고 싶을 때 Batch를 이용합니다. tf.train.batch() 를 통해 함수를 호출할 수 있습니다.
tf.train.batch()
[show_more more=”더 읽기” less=”닫기”]
batch( tensors, batch_size, num_threads=1, capacity=32, enqueue_many=False, shapes=None, dynamic_pad=False, allow_smaller_final_batch=False, shared_name=None, name=None )
Args:
– tensors
: enqueue할 Tensor의 List 혹은 Dictionary 형태
– batch_size
: Queue에서 꺼내올 batch의 크기
– num_threads
: tensors
를 enqueue할 thread의 개수 (* The batching will be nondeterministic if num_threads > 1
. ?)
– capacity
: Queue에 속할 element의 최대 개수(정수형)
– 나머지는 나중에…
Returns:
– 입력 값으로 준 tensors
와 동일 형태의 tensor를 포함하는 List 또는 Dictionary (단 하나의 원소만이 입력되었다면 list가 아닌 하나의 tensor로 나온다고 하네요.)
[/show_more]
Epoch ::
Epoch는 전체 데이터를 학습하는 횟수를 의미합니다. 즉, “1 Epoch = 전체 데이터 학습”을 의미합니다.
Training epoch/batch ::
훈련 데이터가 1,000개 있고 batch_size = 200 이라고 가정해봅시다. 1 Epoch 를 달성하기 위해서는 batch를 5번 반복(Iteration) 해야 합니다. 5번의 반복을 거쳐 모든 데이터를 읽어내면 1 Epoch가 되는 것입니다. 그렇다면 5 Epoch 는 반복 몇번을 의미할까요? 1 Epoch 가 5 반복이므로 5 Epoch 는 25 반복을 의미할 것입니다.
실습
import tensorflow as tf from tensorflow.examples.tutorials.mnist import input_data mnist = input_data.read_data_sets("MNIST_data/", one_hot = True) nb_classes = 10 X = tf.placeholder(tf.float32, [None, 784]) Y = tf.placeholder(tf.float32, [None, nb_classes]) W = tf.Variable(tf.random_normal([784, nb_classes]), name = "weight") b = tf.Variable(tf.random_normal([nb_classes]), name = "bias") scores = tf.matmul(X, W) + b hypothesis = tf.nn.softmax(scores) cost = tf.reduce_mean(-tf.reduce_sum(Y*tf.log(hypothesis), axis=1)) train = tf.train.GradientDescentOptimizer(learning_rate=0.5).minimize(cost)
이전 내용과 같은 Softmax classification 이기 때문에 코드가 매우 흡사합니다. MNIST dataset 은 많은 곳에서 예시로 활용되고 있기 때문에 2번째 줄과 같이 쉽게 불러올 수 있습니다. 불러온 Dataset을 mnist 노드에 담습니다. 한가지 주목해야 할 점은 4번 째 줄에서 mnist 노드에 담을 때 one_hot의 값을 True로 주게 되면 자동으로 One-Hot-Encoding이 되어 노드에 담긴다는 점입니다. <fn>http://pythonkim.tistory.com/46</fn> 위의 코드에서는 데이터를 불러오고 X, Y placeholder 및 W, b 변수를 설정하여 가설 및 train 까지 마쳐놓은 상태입니다.
MNIST 데이터셋 이해하기 : http://pythonkim.tistory.com/46
is_correct = tf.equal(tf.argmax(Y, 1), tf.argmax(hypothesis, 1)) accuracy = tf.reduce_mean(tf.cast(is_correct, tf.float32))
is_correct는 실제값(Y)와 hypothesis의 디코딩 값이 같은지 여부를 담고 있습니다. accuracy는 is_correct의 평균을 구하게 될 op입니다.
training_epoch = 15 batch_size = 100
세션 생성에 앞서 epoch와 batch의 값을 정하도록 하겠습니다.
with tf.Session() as sess: sess.run(tf.global_variables_initializer()) # 같은 데이터로 총 15회 학습시키겠습니다. for epoch in range(training_epoch): # cost의 값을 알아보기 위해 변수를 만들었습니다. avg_cost = 0 # 1 Epoch를 돌기위한 전체 반복 횟수를 결정합니다. total_iteration = int(mnist.train._num_examples / batch_size) for step in range(total_iteration): # mnist dataset에서 batch_size만큼 데이터를 가져와 학습을 시킵니다. batch_xs, batch_ys = mnist.train.next_batch(batch_size) cost_val, _ = sess.run([cost, train], feed_dict={X: batch_xs, Y: batch_ys}) # 학습의 결과인 cost_val을 알아보기 위해 avg_cost에 담습니다. avg_cost += (cost_val / total_iteration) print("Epoch: ", epoch, "cost: ", avg_cost)
* 출력값
Epoch: 0 cost: 1.27970556338
Epoch: 1 cost: 0.615424255878
Epoch: 2 cost: 0.514329390472
Epoch: 3 cost: 0.460816782117
Epoch: 4 cost: 0.427634886002
Epoch: 5 cost: 0.40280714837
Epoch: 6 cost: 0.384523223937
Epoch: 7 cost: 0.369469466399
Epoch: 8 cost: 0.357394137504
Epoch: 9 cost: 0.348266039247
Epoch: 10 cost: 0.33824520531
Epoch: 11 cost: 0.332139841427
Epoch: 12 cost: 0.324647610892
Epoch: 13 cost: 0.319740091251
Epoch: 14 cost: 0.313318256709
print("Accuracy: ", accuracy.eval(feed_dict={X: mnist.test.images, Y: mnist.test.labels}))
* 출력값
Accuracy: 0.9075
MNIST dataset에는 테스트용 이미지가 담겨 있습니다. 여기서 accuracy.eval()의 의미는 sess.run(accuracy)의 의미와 같습니다.
class tf.Tensor
This class has two primary purposes:
A Tensor
can be passed as an input to another Operation
. This builds a dataflow connection between operations, which enables TensorFlow to execute an entire Graph
that represents a large, multi-step computation.
After the graph has been launched in a session, the value of the Tensor
can be computed by passing it totf.Session.run
. t.eval()
is a shortcut for calling tf.get_default_session().run(t)
. <fn>https://www.tensorflow.org/api_docs/python/tf/Tensor</fn>